Table of Contents
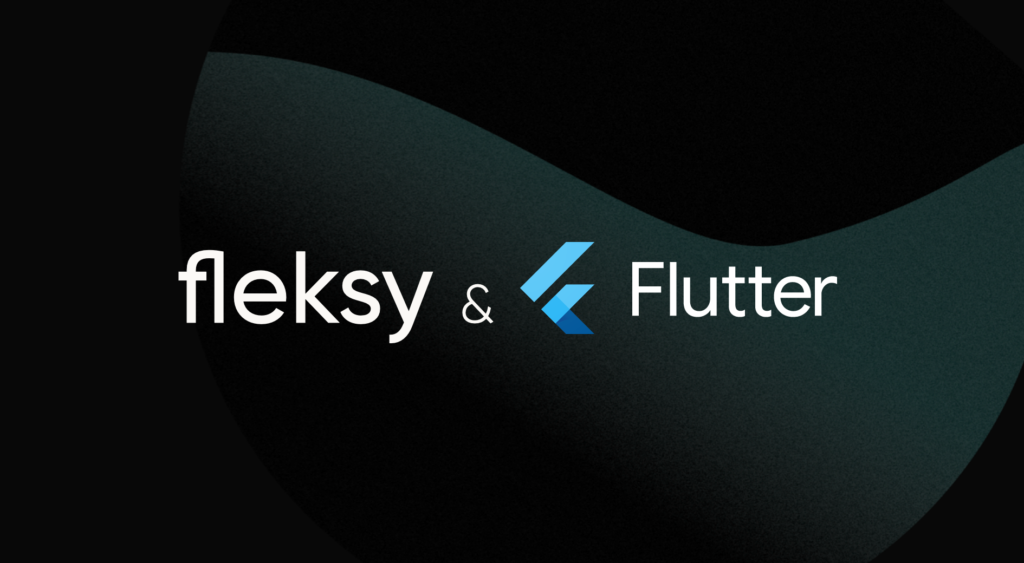
Want to create a custom keyboard app? Combining Flutter with the Fleksy SDK lets you build feature-rich, cross-platform keyboards that are fast, customizable, and privacy-focused.
Key Highlights:
- Platform Support: Develop for iOS, Android, web, and desktop with Flutter.
- Fleksy Features:
- Predictive text, autocorrect, and swipe typing.
- Supports 82 languages with on-device processing for privacy.
- Fully customizable themes and layouts.
- Setup Requirements:
- Flutter SDK, IDE (e.g., Android Studio or VS Code), and Git.
- Fleksy SDK (starts at $269/month with a 30-day free trial).
- Platform-specific tools: Xcode for iOS and Android Studio for Android.
What You’ll Learn:
- How to integrate the Fleksy SDK into your Flutter app.
- Steps to customize keyboard themes, actions, and language support.
- Tips for optimizing performance and ensuring privacy compliance.
This guide walks you through setting up your project, configuring the SDK, and tailoring the keyboard to meet user needs. Let’s get started!
Before You Start
Tools You’ll Need
To get started, make sure you have the following tools ready:
- Flutter SDK: Install the latest stable version for cross-platform app development [3].
- IDE: Use either Android Studio or Visual Studio Code, both with Flutter plugins installed.
- Git: Essential for version control and managing packages.
- Fleksy SDK: Download the package that matches your project’s requirements.
- Platform-Specific Tools:
- For iOS: Xcode 14+ and CocoaPods.
- For Android: Android Studio with necessary SDK tools.
Skills You Should Have
Here’s a quick overview of the knowledge you’ll need:
Skill Category | What You Should Know |
---|---|
Programming | Dart, Object-Oriented Programming |
Flutter | Widgets, State Management, Platform Channels |
Development | API Integration, Package Management |
Platform-Specific | Basics of iOS and Android Development |
Make sure you’re comfortable with these skills before diving in.
Setting Up Your Fleksy Account
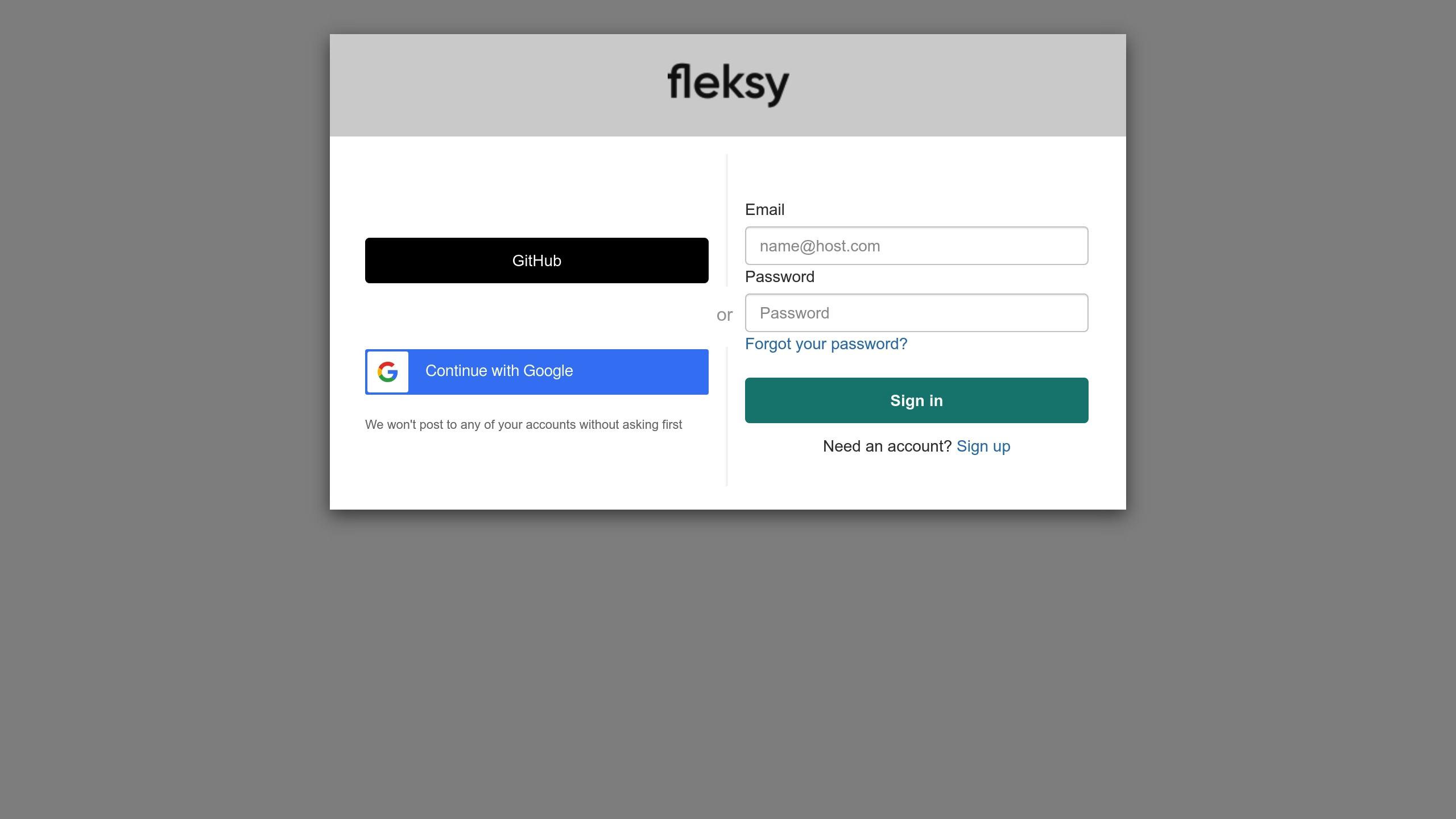
Follow these steps to configure your Fleksy developer environment:
- Sign Up: Visit the Fleksy developer portal and register. The Indie/Solo Plan starts at $269/month and offers features like autocorrect, autocompletion, and swipe input for one app. We suggest you contact Fleksy to confirm your plan is the right one for you.
- Get Your License Key: Log in to the developer dashboard to request your license key [4]. Save this key as an environment variable for easy access.
- Choose Your SDK:
- The Virtual Keyboard SDK is perfect for creating a full keyboard app (iOS/Android).
- The Predictive Text SDK focuses on text prediction across 82 languages (Cross Platform).
Fleksy offers a 30-day free trial, so you can explore its features before committing.
Once you’ve completed these steps, you’re ready to move on to setting up your project.
Related video from YouTube
Project Setup
Ready to start building your keyboard with Fleksy? Here’s how to set up your Flutter project for keyboard development.
New Flutter Project
First, create a new Flutter project by running:
flutter create fleksy_keyboard
cd fleksy_keyboard
Open the project in your IDE and make sure it runs smoothly on both iOS and Android devices. Test this by running:
flutter run
You should see the default counter app. Once confirmed, it’s time to configure the necessary SDK dependencies for both Android and iOS.
SDK Dependencies
Android Configuration
Add the following to your android/build.gradle
file:
allprojects {
repositories {
maven {
url "https://maven.fleksy.com/maven"
}
}
}
Next, update android/app/build.gradle
with this dependency:
dependencies {
implementation 'co.thingthing.fleksycore:fleksycore-release:4.16.0'
}
android {
aaptOptions {
noCompress 'json', 'wav'
}
}
iOS Configuration
To integrate the Fleksy SDK on iOS, use Swift Package Manager:
- Open your project in Xcode.
- Select your app target under “TARGETS”.
- Navigate to “Package Dependencies”.
- Click the “+” button.
- Enter the following URL:
https://github.com/FleksySDK/FleksySDK-iOS
.
Once these dependencies are set, you’re ready for platform-specific configurations.
Platform Setup
Platform | Required Setup | Configuration File |
---|---|---|
Android | Android SDK settings, Minimum SDK 21 | android/app/build.gradle |
iOS | Custom Keyboard Extension, Permissions | Info.plist |
Android Setup
- Create an assets directory for encrypted files:
mkdir -p android/app/src/main/assets/encrypted
- Add the English language pack:
cp resourceArchive-en-US.jet android/app/src/main/assets/encrypted/
iOS Setup
- Add a new Keyboard Extension target in Xcode.
- Update the extension’s
Info.plist
to include the required permissions. - Disable Bitcode if you’re using Xcode versions older than 14.
SDK Implementation
This section explains how to integrate the Fleksy SDK into your Flutter app, starting from project configuration to platform-specific setup.
SDK Setup
Start by creating a Dart file (keyboard_service.dart
) to handle communication with the native SDK:
class KeyboardService {
static const platform = MethodChannel('com.your.app/keyboard');
Future<void> initializeSDK(String licenseKey) async {
try {
await platform.invokeMethod('initializeKeyboard', {
'licenseKey': licenseKey,
});
} catch (e) {
print('Error initializing keyboard: $e');
}
}
}
Store your Fleksy license key securely as an environment variable:
final licenseKey = const String.fromEnvironment('FLEKSY_LICENSE_KEY');
Once the SDK is initialized, you’ll need to configure the required permissions for both Android and iOS.
Permission Setup
Android: Add the keyboard service permission to your AndroidManifest.xml
file:
<service
android:name=".SampleKeyboardService"
android:directBootAware="true"
android:exported="true"
android:permission="android.permission.BIND_INPUT_METHOD">
<intent-filter>
<action android:name="android.view.InputMethod" />
</intent-filter>
<meta-data
android:name="android.view.im"
android:resource="@xml/sample_input_method" />
</service>
iOS: Update the required permissions in your Info.plist
file and system settings:
Setting | Location | Value |
---|---|---|
RequestsOpenAccess | Info.plist | YES |
Privacy – Input Method | Info.plist | Required for keyboard functionality |
Full Access | Settings > Keyboard | Enable |
Platform Code
After setting permissions, implement the platform-specific code for Android and iOS.
Android: Create a Kotlin class in android/app/src/main/kotlin
:
class FleksyKeyboardService : KeyboardService() {
override fun createConfiguration(): Configuration {
return Configuration.Builder()
.setLicenseKey(BuildConfig.FLEKSY_LICENSE_KEY)
.build()
}
}
iOS: Implement the keyboard extension using Swift:
import FleksyKeyboardSDK
class KeyboardViewController: FKKeyboardViewController {
override func createConfiguration() -> Configuration {
return Configuration.Builder()
.setLicenseKey(ProcessInfo.processInfo.environment["FLEKSY_LICENSE_KEY"])
.build()
}
}
With these steps, your app will be ready to use the Fleksy SDK effectively on both platforms.
Keyboard Customization
Once the core SDK setup is in place, the next step is to personalize your keyboard’s appearance and functionality. You can adjust themes, add custom actions, and enable multilingual support to create a tailored user experience.
Visual Settings
The Fleksy SDK makes it easy to customize the keyboard’s appearance on both Android and iOS. Here’s how you can update the theme using Dart:
Future<void> setKeyboardTheme(ThemeConfig config) async {
try {
await platform.invokeMethod('updateTheme', {
'backgroundColor': config.backgroundColor,
'keyColor': config.keyColor,
'textColor': config.textColor,
'fontFamily': config.fontFamily
});
} catch (e) {
print('Error updating theme: $e');
}
}
For Android, you can define the style configuration like this:
override fun configureStyle(): StyleConfiguration {
return StyleConfiguration.Builder()
.setKeyboardBackground("#FFFFFF")
.setKeyBackground("#F0F0F0")
.setKeyTextColor("#000000")
.setFontFamily("Roboto")
.build()
}
For iOS, the equivalent Swift code looks like this:
override func configureStyle() -> StyleConfiguration {
return StyleConfiguration.Builder()
.setKeyboardBackground("#FFFFFF")
.setKeyBackground("#F0F0F0")
.setKeyTextColor("#000000")
.setFontFamily("SF Pro")
.build()
}
Custom Functions
You can extend the keyboard’s capabilities by adding custom shortcuts or actions. Here’s an example in Dart:
class CustomKeyboardActions {
static const platform = MethodChannel('com.your.app/keyboard_actions');
Future<void> addCustomShortcut(String trigger, String expansion) async {
await platform.invokeMethod('addShortcut', {
'trigger': trigger,
'expansion': expansion
});
}
}
This allows you to define specific triggers and their corresponding expansions, enhancing the keyboard’s utility.
Language Options
To enable multilingual support, use the LanguageConfiguration
class. Here’s a Dart example:
Future<void> configureLanguages(List<String> languages) async {
final languageConfig = {
'userLanguages': languages,
'automaticDownload': true,
'current': 'en-US'
};
await platform.invokeMethod('setLanguageConfig', languageConfig);
}
You can also customize the spacebar to display a logo, active language, or switch dynamically based on context:
Spacebar Style | Description | Ideal For |
---|---|---|
.Automatic | Switches between language or logo depending on context | Apps supporting multiple languages |
.LogoOnly | Displays only the Fleksy logo | Single-language setups |
.LanguageOnly | Shows the active language identifier | Language-centric applications |
For Android, configure languages like this:
override fun createLanguageConfig(): LanguageConfiguration {
return LanguageConfiguration.Builder()
.setUserLanguages(arrayOf("en-US", "es-ES"))
.setAutomaticDownload(true)
.build()
}
For iOS, use the following Swift code:
override func createLanguageConfig() -> LanguageConfiguration {
return LanguageConfiguration.Builder()
.setUserLanguages(["en-US", "es-ES"])
.setAutomaticDownload(true)
.build()
}
Testing Guide
Thorough testing is key to ensuring your keyboard performs well across different devices and use cases. Here’s how to check and fine-tune your Fleksy SDK implementation.
Device Testing
Test your keyboard’s performance on a variety of devices by enabling performance monitoring in profile mode. Focus on:
- Latest smartphones: Test on current iPhone and Android models in both portrait and landscape modes.
- Older devices: Include models like iPhone 11 or Pixel 4 to catch issues on less powerful hardware.
- Tablets: Test on devices such as iPads or Samsung Tablets.
- Low-memory environments: Simulate scenarios where memory is limited.
Here’s an example of enabling performance monitoring:
void main() {
enablePerformanceOverlay();
runApp(MyKeyboardApp());
}
This helps you spot and address performance issues early.
Fixing Common Issues
If you encounter problems like input lag or slow rendering, check whether latency exceeds 16ms. This is the threshold for smooth 60 FPS rendering. Use the following diagnostic tool:
class KeyboardDebugger {
static Future<void> diagnoseInputLag() async {
final performance = await platform.invokeMethod('getInputMetrics');
if (performance['inputLatency'] > 16.0) {
log('Input lag detected; implement lag reduction strategies');
}
}
}
Flutter DevTools is a great resource here. Use it to track input latency, memory usage during language switches, and rendering times for keyboard layouts. Once you’ve identified the problem, optimize accordingly.
Boosting Performance
Improve your keyboard’s speed and responsiveness by using Flutter’s Performance Overlay and DevTools. Keep an eye on:
- UI Thread: Tracks Dart code execution.
- Raster Thread: Monitors GPU rendering tasks.
To maintain smooth performance on 60 FPS devices, ensure each frame renders in under 16ms. Use the Timeline view in DevTools to examine performance frame-by-frame.
For benchmarking, use Flutter Driver to measure performance metrics:
Future<void> benchmarkKeyboard() async {
final timeline = await driver.traceAction(() async {
await driver.tap(find.byType('KeyboardView'));
await driver.enterText('performance test');
});
final summary = TimelineSummary.summarize(timeline);
await summary.writeTimelineToFile('keyboard_benchmark');
}
Profile Mode Insights
Profile mode offers debugging tools while maintaining performance close to a release build. Use it to monitor:
- Frame rendering times
- Text prediction speed
- Memory usage patterns
- Widget rebuilds
These metrics will help ensure your keyboard feels responsive and efficient across all devices.
Conclusion
With implementation and testing wrapped up, let’s recap the highlights.
Key Points
The Fleksy SDK can save developers a huge amount of time – cutting development time by up to 90% [1]. Its streamlined design allows for building feature-packed keyboards without sacrificing speed or performance.
Here are a few key things to keep in mind:
- Platform Configuration: Ensure iOS and Android settings are properly set up.
- Language Support: Take advantage of support for over 80 languages [2].
- Visual Customization: Use theming options to adjust colors, fonts, and backgrounds to suit your app.
Advanced Features
The Fleksy SDK offers extra tools to take your keyboard to the next level:
- Custom Actions: Add unique buttons directly to the keyboard for app-specific functions [2].
- Top Bar Customization: Include custom icons or views on either side of the top bar [2].
- Predictive Technologies: Implement features like:
- Autocorrection
- Next-word prediction
- Swipe typing [6]
These features let you go beyond the basics and create a keyboard tailored to your users’ needs.
Help Resources
Need support? Fleksy offers several resources to assist you:
- Documentation Portal: Find detailed guides and API references.
- GitHub Discussion Forum: Collaborate with other developers and exchange ideas.
- Discord Community: Get quick help from the Fleksy team in real time.
- Developer Portal: Submit technical or business-related questions.
With its customization options and strong support network, the Fleksy SDK is a dependable choice for crafting powerful keyboards that can grow with your app.