Table of Contents
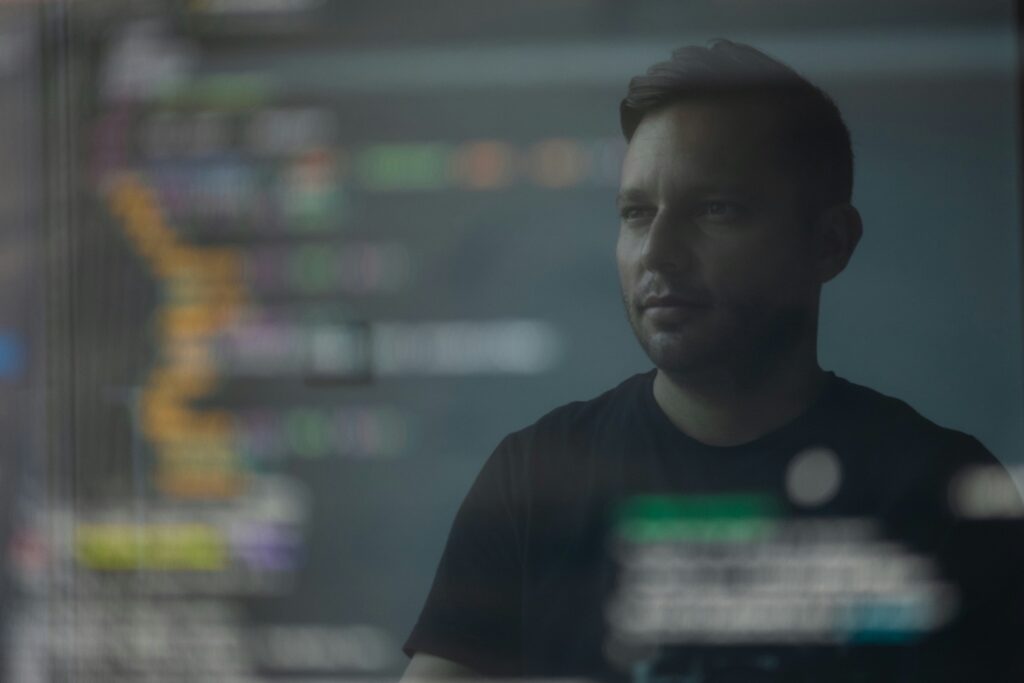
Autocorrect has become an essential feature in modern keyboard apps, enhancing user experience by minimizing typing errors and speeding up communication. If you’re developing a custom Android keyboard, incorporating autocorrect functionality can set your app apart. In this comprehensive guide, we’ll walk you through the process of adding autocorrect to your keyboard app and introduce you to the Fleksy Predictive Text SDK, a tool that can streamline your development process.
Introduction
Creating a custom keyboard app offers endless possibilities for innovation, but implementing advanced features like autocorrect can be complex. Autocorrect enhances user satisfaction by automatically correcting typos and suggesting words, making your app more intuitive and user-friendly.
Understanding Autocorrect Mechanisms
Autocorrect works by comparing the words a user types against a comprehensive dictionary. When a typo is detected, the system suggests the most likely correct word based on various algorithms and linguistic data.
Key Components of Autocorrect:
- Dictionary Database: A comprehensive list of words and phrases.
- Error Detection Algorithms: Identify typos and mismatches.
- Correction Suggestions: Offer alternative words based on context and likelihood.
Setting Up Your Development Environment
Before diving into code, ensure you have the necessary tools:
- Android Studio: The official IDE for Android development.
- Programming Language: Kotlin or Java (Kotlin is recommended for modern Android apps).
- Android SDK: Ensure it’s updated to the latest version.
Implementing Autocorrect from Scratch
Building autocorrect involves several steps, each requiring careful attention to detail.
Building a Dictionary
Your autocorrect system needs a robust dictionary.
Steps to Build a Dictionary:
- Collect Data: Use open-source word lists or compile your own.
- Optimize Storage: Implement efficient data structures like tries or hash maps.
- Include Frequencies: Store the frequency of each word’s usage to improve suggestion accuracy.
Code Example in Kotlin:
val dictionary = HashMap<String, Int>()
dictionary["example"] = 5000
dictionary["sample"] = 3000
Error Detection Algorithms
Detecting typos is crucial for autocorrect.
Common Algorithms:
- Levenshtein Distance: Measures the difference between two words.
- N-Grams: Analyzes sequences of characters or words.
- Bayesian Probability: Predicts the likelihood of word sequences.
Implementing Levenshtein Distance:
fun levenshtein(a: String, b: String): Int {
val costs = IntArray(b.length + 1)
for (j in 0..b.length) costs[j] = j
for (i in 1..a.length) {
costs[0] = i
var nw = i - 1
for (j in 1..b.length) {
val cj = minOf(
1 + costs[j],
1 + costs[j - 1],
if (a[i - 1] == b[j - 1]) nw else nw + 1
)
nw = costs[j]
costs[j] = cj
}
}
return costs[b.length]
}
Suggesting Corrections
Once errors are detected, suggest the most probable corrections.
Steps:
- Generate Candidates: Find words in the dictionary similar to the misspelled word.
- Rank Candidates: Use word frequency and context to rank suggestions.
- Display Suggestions: Show the top suggestions to the user.
Code Snippet:
codefun suggest(word: String): List<String> {
return dictionary.keys.filter {
levenshtein(it, word) <= 2
}.sortedBy {
dictionary[it]
}.take(3)
}
Challenges in Autocorrect Implementation
Implementing autocorrect is resource-intensive.
- Performance Issues: Real-time suggestions require optimized code.
- Language Support: Multilingual support adds complexity.
- Contextual Accuracy: Understanding user intent is challenging.
Simplifying the Process with Fleksy Predictive Text SDK
The Fleksy Predictive Text SDK offers a robust solution to these challenges, providing advanced autocorrect features out of the box.
Benefits of Using Fleksy Predictive Text SDK
- Advanced Autocorrect Engine: Leverages years of development and data.
- Multilingual Support: Supports over 80 languages.
- Easy Integration: Simple API to add autocorrect to your app.
- Customizable: Tailor the keyboard experience to your app’s needs.
- Performance Optimized: Efficient algorithms ensure smooth user experience.
How to Integrate Fleksy Predictive Text SDK into Your App
Step 1: Sign Up to use one of our Fleksy SDKs
- Visit the Fleksy SDK website and sign up for access.
Step 2: Add the Predictive Text SDK to Your Project
- Include the Fleksy Predictive Text SDK in your project’s dependencies.
Gradle Example:
dependencies {
implementation 'com.fleksy.sdk:fleksy-sdk:_version_'
}
Step 3: Initialize the SDK
Fleksy.initialize(context, "YOUR_API_KEY")
Step 4: Configure Autocorrect
Customize autocorrect settings to suit your app.
codeval fleksySettings = FleksySettings.Builder()
.enableAutocorrect(true)
.build()
Fleksy.configure(fleksySettings)
Step 5: Implement the Keyboard View
Add the Fleksy keyboard to your layout.
<com.fleksy.sdk.keyboard.FleksyKeyboardView
android:id="@+id/fleksyKeyboard"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
Step 6: Handle User Input
Use the Fleksy SDK’s callbacks to manage text input.
fleksyKeyboard.setOnTextChangedListener { text ->
// Handle the text input
}
Conclusion
Adding autocorrect functionality to your custom Android keyboard significantly enhances user experience but can be complex to implement from scratch. While building your own autocorrect engine is a valuable learning experience, it often involves challenges like performance optimization and multilingual support.
The Fleksy SDK simplifies this process, offering a powerful, ready-to-use autocorrect engine with easy integration and extensive customization options. By leveraging the Fleksy SDK, you can focus on innovating other aspects of your keyboard app, reducing development time, and delivering a superior product to your users.
Ready to Enhance Your Keyboard App with Advanced Autocorrect?
Take your custom Android keyboard to the next level with the Fleksy Predictive Text SDK. Get started with Fleksy SDK today and provide your users with an unparalleled typing experience.