Table of Contents
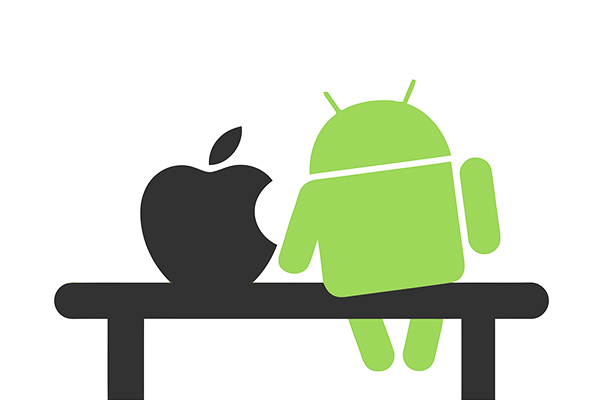
Custom keyboard apps are a popular feature, and integrating a Keyboard SDK can simplify the development process. Here’s a quick look at how iOS and Android differ when it comes to integrating these SDKs:
- Development Tools: iOS uses Xcode with Swift/Objective-C, while Android relies on Android Studio with Java/Kotlin.
- Integration Frameworks: iOS supports custom keyboard extensions, whereas Android uses the Input Method Editor (IME) system.
- Setup Time: iOS offers a streamlined setup process with Swift Package Manager, while Android provides more flexibility through Maven and Gradle.
- Customization: iOS emphasizes app-level integration, while Android allows for deeper system-level customization.
- Performance: iOS apps are smaller in size and use less memory, but Android offers more control over system resources.
- Security: Both platforms enforce strict security and privacy guidelines, but implementation methods differ.
Quick Comparison
Aspect | iOS | Android |
---|---|---|
Development Tools | Xcode, Swift/Objective-C | Android Studio, Java/Kotlin |
Integration Framework | Custom Keyboard Extension | Input Method Editor (IME) |
Package Management | Swift Package Manager | Maven |
Setup Complexity | Simple, guided process | Flexible, customizable process |
Customization | App-level integration | System-level customization |
Performance | Smaller app size, lower memory usage | More control over resources |
Security | Sandbox environment, strict permissions | Secure storage, granular permissions |
This article dives deeper into the setup, design, performance, and security aspects of building custom keyboards for iOS and Android.
Fleksy Keyboard iOS SDK – Quick Starter Guide for building virtual keyboard applications for iOS
Development Setup
To successfully integrate a keyboard SDK, it’s essential to properly set up your development environment. The tools and configurations needed vary between iOS and Android platforms.
Platform | Core Tools | Key Components |
---|---|---|
iOS | Xcode, Swift/Objective-C | Keyboard templates, package management, simulator |
Android | Android Studio, Java/Kotlin | Gradle dependencies, emulators, debugging tools |
iOS Development Environment
For iOS, Xcode is your go-to tool, with Swift being the primary programming language. The latest version (15.2 as of February 2025) requires setting up specific permissions and entitlements for keyboard extensions. Xcode simplifies the process with built-in templates and the Swift Package Manager, making SDK integration straightforward. Plus, the iOS Simulator lets you thoroughly test your keyboard before deployment.
Android Development Environment
On Android, Android Studio is the main development platform. Tools like Gradle handle dependencies, and emulators provide a reliable way to test your keyboard. Adding a keyboard SDK is as simple as including a line in the build.gradle file through Maven [1]. Android Studio also offers features for managing dependencies, testing, and UI debugging.
Development Resources
Both platforms provide extensive documentation and tools to assist with SDK integration. iOS developers can find centralized resources within Xcode and Apple’s developer portal. Android developers benefit from guides, forums, and tools available on the Android developer site [1][2]. These materials help ensure your keyboard works smoothly across various devices and OS versions.
Once your environment is ready, the next step is configuring the keyboard SDK for your platform.
Setup Steps
Setting up keyboard SDKs varies between iOS and Android platforms. Here’s a breakdown of how to implement them on each operating system.
iOS Keyboard Setup
For iOS, the setup process uses Xcode’s extension features with Swift or Objective-C:
Setup Component | iOS Implementation Details |
---|---|
Project Configuration | Create a custom keyboard extension target in Xcode |
Required Permissions | Enable Full Access entitlement and add necessary privacy declarations |
Integration Method | Use Swift Package Manager for dependency management |
Core Components | Input view controller (handles keyboard interface), extension delegate |
To get started, create a custom keyboard extension target in Xcode. Then, set up permissions and entitlements, such as Full Access, to ensure proper functionality. Implement the InputViewController
class to handle the keyboard’s interface and use Swift Package Manager to manage dependencies. This method focuses on app-level integration through extensions [1].
Android IME Setup
For Android, the process relies on Android Studio and the platform’s Input Method Editor (IME) framework:
Setup Component | Android Implementation Details |
---|---|
Project Setup | Configure Gradle and declare the IME service in the manifest |
System Integration | Implement the InputMethodService class |
Dependencies | Add required libraries via Maven |
Core Components | IME service, input view (manages keyboard layout), keyboard layout |
To integrate the SDK on Android, start by configuring Gradle and declaring the IME service in the app’s manifest. Implement the InputMethodService
class to handle system-level input functionality. Gradle dependencies can be managed through Maven, providing the necessary libraries for your project. While the process involves more configuration, it allows for deeper system-level integration [1][2].
iOS focuses on a simpler, app-based setup, while Android offers more extensive system integration, reflecting their differing architectures and security models.
With the SDK set up, you can now dive into customizing and designing your keyboard for a tailored user experience.
Design Options
When building keyboard apps, iOS and Android each take a different path when it comes to customizing visuals and implementing features. Here’s a closer look at what each platform offers.
Visual Customization
Both platforms allow a range of options for tweaking the keyboard’s look, but they go about it in distinct ways:
Feature | iOS Implementation | Android Implementation |
---|---|---|
Theme Support | Custom themes via UIKit extensions | XML-based themes with the IME framework |
Layout Control | Interface Builder or programmatic UI | Layout XML files with custom views |
Custom Views | Top bar customization using UIKit components | Floating views and overlays supported |
Orientation Handling | Auto-layout for dynamic orientation changes | Android-specific layout resources |
Language Support
Support for multiple languages is a key feature, with up to 82 languages available. However, iOS and Android handle this in different ways:
Aspect | iOS | Android |
---|---|---|
Language Switch | System-level language picker | In-keyboard language selector |
Input Methods | Uses the native input method framework | Built on InputMethodService |
Character Sets | Unicode handled via NSString | Managed by Android’s text system |
Regional Variants | Locale-based customizations | Resource qualifiers for localization |
Extra Features
Beyond looks and language, both platforms provide tools to improve the keyboard’s functionality and user experience:
Feature Type | Details |
---|---|
Text Prediction & Autocorrection | Uses platform-specific machine learning algorithms |
Custom Actions | Enables API-driven button functionality |
Gesture Support | Built with touch event handling systems |
Apps like BlindSquare and Launch Center Pro have successfully used these features to create tailored keyboard solutions for their users [3].
Balancing design flexibility with efficient coding is key to ensuring smooth performance. While customization is important, technical optimization ensures these features work seamlessly in everyday use.
Technical Performance
When adding keyboard SDKs to an app, how well they perform directly affects how smooth and reliable the app feels to users.
Resource Usage and Speed
Keyboard SDKs influence app size, memory usage, and other performance aspects differently on iOS and Android. Here’s a breakdown of key metrics:
Metric | iOS | Android |
---|---|---|
Base App Size | 2-5 MB (Swift Package) | 1-3 MB (Maven Package) |
Active Memory | 215-30 MB | 20-40 MB |
Background Process | Minimal (system-managed) | Service-based consumption |
Cache Requirements | Local prediction data | Shared IME resources |
Input Latency | Direct UIKit integration | InputMethodService pipeline |
Layout Loading | Interface Builder caching | XML layout inflation |
Thread Management | Main thread UI updates | Background service handling |
Developer Experience
Creating a responsive keyboard experience depends on how easily developers can work with the SDK. iOS and Android each present unique challenges during implementation.
Development Factor | iOS | Android |
---|---|---|
Setup Time | 2-3 days average | 3-4 days average |
Testing Requirements | TestFlight validation | Play Store review |
Documentation Access | Apple Developer Portal | Android Developers site |
Debug Tools | Xcode Instruments | Android Studio Profiler |
“Key challenges include resource management, device compatibility, and platform-specific requirements” [1][4].
Once performance is optimized, the next focus should be meeting security standards specific to each platform.
Security Standards
Security is just as important as performance when it comes to creating a reliable keyboard experience.
Data Security
Both iOS and Android offer strong frameworks to keep keyboard input data secure:
Security Aspect | iOS | Android |
---|---|---|
Encryption Standard | Enforced encryption standards | Android KeyStore system |
Data Storage | Local sandbox environment | Secure shared storage |
Input Protection | CoreText secure input fields | InputMethodService security |
Sensitive Data Handling | No local storage permitted | Encrypted temporary cache |
Authentication | App-specific keyboard container | IME service verification |
Platform Rules
Both platforms enforce strict security guidelines for keyboard SDKs:
Requirement | iOS Guidelines | Android Guidelines |
---|---|---|
Review Process | App Store security validation | Play Store safety checks |
Permission Model | Explicit keyboard access | Granular IME permissions |
Update Compliance | Regular security patches | Quarterly security updates |
Data Collection | Limited user data gathering | Transparent data policies |
Network Access | Restricted connectivity | Controlled API access |
These rules are the foundation for ensuring privacy and security in keyboard apps.
Privacy Protection
Protecting user privacy during keyboard use involves multiple safeguards. For instance, iOS enforces strict protocols through its App Store Review Guidelines [1], while Android relies on secure storage via the Android KeyStore system [4].
Key privacy features include:
Privacy Feature | iOS Implementation | Android Implementation |
---|---|---|
Input Protection & Transmission | System-level field protection with end-to-end encryption | Custom input filtering with TLS enforcement |
User Consent | Granular privacy settings | User-controlled permissions |
Analytics Collection | Anonymized usage data | Aggregated statistics only |
Cloud Sync | Optional encrypted backup | User-controlled sync |
“Failure to meet security guidelines risks app rejection and legal penalties” [1].
These measures not only comply with platform standards but also build user confidence in the security of their keyboard interactions.
Conclusion
Platform Differences
Developing for iOS and Android presents distinct approaches and tools. iOS development relies on Swift or Objective-C within Xcode, offering a controlled setup. On the other hand, Android uses Java or Kotlin in Android Studio, which allows for more customization.
Integration Aspect | iOS Environment | Android Environment |
---|---|---|
Package Management | Swift Package Manager | Maven integration |
Integration Complexity | Guided, structured process | Flexible, customizable approach |
Platform Support | Fewer device variations | Wide range of device configurations |
These differences shape how developers approach integration and the strategies they employ.
Developer Guidelines
When deciding on a platform for keyboard SDK integration, take these factors into account:
Decision Factor | iOS Consideration | Android Consideration |
---|---|---|
Time to Market | Streamlined review process | Quicker deployment cycle |
Development Ecosystem | Unified tools and resources | Broad toolset and community |
Market Reach | Smaller but high-value users | Broader user base |
Maintenance Effort | Predictable update cycles | Varies across devices |
“The differences in setup steps, design options, and technical performance can significantly impact the developer experience” [1].
To achieve the best results, developers should focus on these steps:
- Evaluate Project Needs: Align platform features with your technical requirements.
- Assess Resource Availability: Ensure the team and tools fit the platform’s demands.
- Plan for Updates: Factor in security patches and platform-specific update cycles.
FAQs
How to create a keyboard app for iOS?
To build a keyboard app for iOS, start by opening your project in Xcode. From there, navigate to File > New > Target and choose the Custom Keyboard Extension option. You’ll need to set up details like the extension’s name, language, and permissions. Once configured, Xcode will automatically include the extension in your app bundle.
Xcode’s environment makes the setup process straightforward, emphasizing app-level integration. To manage dependencies efficiently, you can use the Swift Package Manager, as explained in the Development Setup section.
This method showcases iOS’s strong focus on app-level integration, which differs from Android’s system-level customization process. iOS simplifies the process, while Android offers more room for deeper system-level adjustments.