Table of Contents
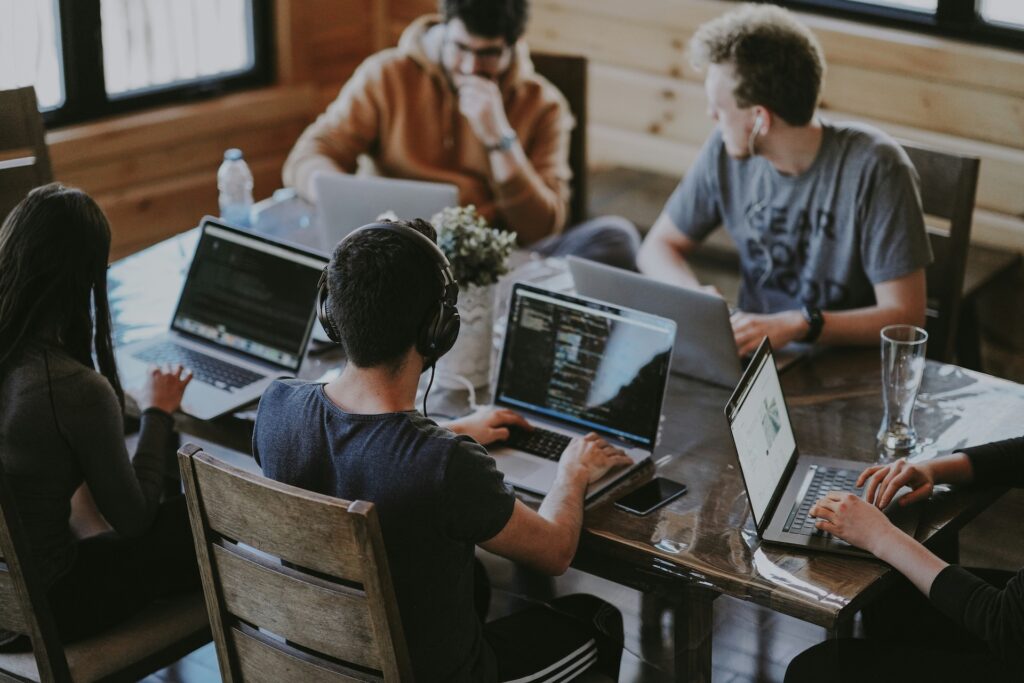
Creating a custom keyboard for iOS opens up a world of possibilities to enhance user experience and introduce innovative features. However, with this opportunity comes the responsibility of ensuring that your keyboard app is secure and respects user privacy. Apple’s strict guidelines and users’ growing concerns about data security make it imperative to prioritize security features during development.
In this comprehensive guide, we’ll delve into the essential security considerations when building an iOS custom keyboard. We’ll also explore how the Fleksy SDK can simplify the development process while ensuring your keyboard app meets the highest security standards.
1. Understanding Apple’s Security Guidelines
Before you start developing your custom keyboard, it’s crucial to familiarize yourself with Apple’s App Store Review Guidelines, particularly those related to keyboard extensions.
Key Points:
- User Privacy: Apps must obtain user consent before accessing personal data.
- Data Minimization: Collect only the data necessary for your app’s functionality.
- Full Access: If your keyboard requires “Full Access,” you must explain why and how user data will be used.
- Secure Transmission: Any collected data must be transmitted securely using HTTPS.
- No Third-Party Sharing: User data should not be shared with third parties without explicit consent.
Understanding these guidelines ensures that your app complies with Apple’s policies, reducing the risk of rejection during the App Store review process.
2. Avoiding Unnecessary Full Access Requests
Requiring “Full Access” can raise red flags for users concerned about privacy. Full Access allows the keyboard to communicate with its container app and the internet, which could potentially expose sensitive information.
Strategies:
- Limit Functionality to Essential Features: Design your keyboard to function effectively without Full Access when possible.
- Transparent Communication: If Full Access is necessary, provide a clear and concise explanation within the app and App Store description.
- Local Processing: Perform text processing and feature implementation on the device to minimize data transmission.
3. Handling User Data Responsibly
Proper management of user data is at the heart of app security.
Best Practices:
- No Keystroke Logging: Avoid storing or transmitting individual keystrokes.
- Anonymized Data Collection: If you collect usage data, ensure it’s aggregated and anonymized.
- Secure Storage: Use encrypted containers for any stored data within the app.
- Data Retention Policies: Define clear policies for how long data is stored and when it’s deleted.
4. Implementing Secure Communication
If your keyboard app needs to communicate with a server, ensuring the security of this communication is vital.
Measures:
- Use HTTPS Protocols: Always use HTTPS with SSL/TLS encryption for data transmission.
- Certificate Pinning: Implement certificate pinning to prevent man-in-the-middle attacks.
- API Security: Use secure APIs with authentication tokens to protect data endpoints.
5. Disabling Features in Secure Input Fields
Sensitive input fields, such as password fields, require special handling to prevent data leakage.
Actions:
- Detect Secure Fields: Use the
textDocumentProxy.keyboardType
to identify secure input fields. - Disable Predictive Text: Turn off autocorrect and predictive text features in secure fields.
- Prevent Clipboard Access: Ensure your keyboard doesn’t allow copying or pasting in secure fields.
Code Snippet for Disabling Features:
override func textDidChange(_ textInput: UITextInput?) {
let proxy = self.textDocumentProxy
if proxy.keyboardType == .asciiCapableNumberPad || proxy.keyboardType == .numberPad {
// Disable features in secure fields
disableAutocorrect()
} else {
enableAutocorrect()
}
}
6. Preventing Unauthorized Data Access
Protecting user data from unauthorized access within your app is essential.
Strategies:
- Sandboxing: Utilize iOS sandboxing to limit the app’s access to user data and system resources.
- Input Validation: Validate all inputs to prevent injection attacks.
- Regular Audits: Conduct security audits to identify and fix vulnerabilities.
7. Regular Security Updates and Monitoring
Security isn’t a one-time task but an ongoing process.
Steps:
- Stay Informed: Keep up-to-date with the latest security advisories and patches from Apple.
- Update Dependencies: Regularly update third-party libraries and SDKs to their latest secure versions.
- User Notifications: Inform users about significant security updates and encourage them to keep the app updated.
8. Leveraging Fleksy SDK for Enhanced Security
Building a secure keyboard from scratch can be complex and time-consuming. The Fleksy SDK offers a secure, efficient, and customizable solution that simplifies this process.
Benefits of Using Fleksy SDK:
- Compliance with Guidelines: Fleksy SDK is designed to meet Apple’s stringent security and privacy requirements.
- On-Device Processing: All text input processing occurs locally, eliminating unnecessary data transmission.
- No Personal Data Collection: The SDK doesn’t collect or store personal user data, ensuring privacy.
- Easy Integration: Simple to integrate into your app with comprehensive documentation and support.
- Customizable Security Features: Allows you to enable or disable features like autocorrect in secure fields easily.
Integrating Fleksy SDK:
Step 1: Sign Up and Obtain API Key
- Visit the Fleksy SDK website to sign up and get your API key.
Step 2: Install Fleksy SDK
- Use CocoaPods to add Fleksy SDK to your project.
Step 3: Import and Initialize
import FleksySDK
class KeyboardViewController: UIInputViewController {
override func viewDidLoad() {
super.viewDidLoad()
FleksySDK.initialize(apiKey: "YOUR_API_KEY")
}
}
Step 4: Configure Security Settings
- Customize security features as per your requirements.
FleksySDK.shared.enableSecureInputMode = true
FleksySDK.shared.onSecureInputDetected = { isSecureField in
if isSecureField {
FleksySDK.shared.enableAutocorrect(false)
} else {
FleksySDK.shared.enableAutocorrect(true)
}
}
Step 5: Test Thoroughly
- Ensure that all security features function correctly across different scenarios and input fields.
Conclusion
Security is a critical aspect of developing an iOS custom keyboard. By prioritizing security features and adhering to best practices, you protect your users and build trust in your app. Implementing these security measures can be challenging, but leveraging tools like the Fleksy SDK can significantly simplify the process.
The Fleksy SDK not only accelerates development but also ensures your keyboard app meets the highest security standards. By focusing on user privacy and secure data handling, you increase the chances of your app being approved by Apple and embraced by users.
Enhance your keyboard app with robust security features using the Fleksy SDK. Get started with Fleksy SDK today and deliver a secure, high-performance keyboard that users will trust.